步骤条Stepper
Stepper
const Stepper({
super.key,
required this.steps,// List<Step>
this.physics,//滑动的物理效果
this.type = StepperType.vertical,//横向与纵向两种,默认为 StepperType.vertical
this.currentStep = 0,//当前 step,默认为 0
this.onStepTapped,//step 点击回调函数
this.onStepContinue,//Next 按钮点击回调函数
this.onStepCancel,//Cancel 按钮点击回调函数
this.controlsBuilder,//内容下方按钮构建函数
this.elevation,//阴影 仅在Stepper的type为横向时有效
this.margin,//外边距 仅在Stepper的type为垂直时有效
})
steps
const Step({
required this.title,//标题
this.subtitle,//副标题控件
required this.content,// 内容控件
this.state = StepState.indexed,//当前 step 的状态,StepState 会改变每一个 step 的图标,默认为 StepState.indexed
this.isActive = false,//是否激活状态,默认为 false,isActive == true 时会变成蓝色
this.label,//标签,StepperType.horizontal有效
})
基本使用方法
import 'package:flutter/material.dart';
class GRStepperPage extends StatefulWidget {
const GRStepperPage({Key? key}) : super(key: key);
@override
State<GRStepperPage> createState() => _GRStepperPageState();
}
class _GRStepperPageState extends State<GRStepperPage> {
//当前step
int _currentStep = 0;
// 点击下一个步骤
_stepTapped(int step){
setState(() {
_currentStep = step;
});
}
// 继续
_stepContinue(){
if(_currentStep < 2){
setState(() {
_currentStep += 1;
});
}else{
null;
}
}
//取消
_stepCancel(){
if(_currentStep > 0){
setState(() {
_currentStep -= 1;
});
}else{
null;
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('步骤条'),),
body: Padding(
padding: const EdgeInsets.all(15.0),
child: Column(
children: [
Expanded(
child: Stepper(
physics: const ScrollPhysics(),
type: StepperType.horizontal,
currentStep: _currentStep,
onStepTapped: (step) => _stepTapped(step),
onStepContinue: _stepContinue,
onStepCancel: _stepCancel,
steps: [
Step(
// label: const Icon(Icons.person),
title: const Text('姓名'),
content: Column(
children: [
TextFormField(
decoration: const InputDecoration(labelText: '你的名字'),
),
],
),
isActive: _currentStep >= 0,
state: _currentStep >= 0 ? StepState.complete : StepState.disabled
),
Step(
title: const Text('手机号'),
content: Column(
children: [
TextFormField(
decoration: const InputDecoration(labelText: '你的手机号'),
),
],
),
isActive: _currentStep >= 0,
state: _currentStep >= 1 ? StepState.complete : StepState.disabled
),
Step(
title: const Text('地址'),
content: Column(
children: [
TextFormField(
decoration: const InputDecoration(labelText: '你的地址'),
),
],
),
isActive: _currentStep >= 0,
state: _currentStep >= 2 ? StepState.complete : StepState.disabled
),
]
),
)
],
),
),
);
}
}
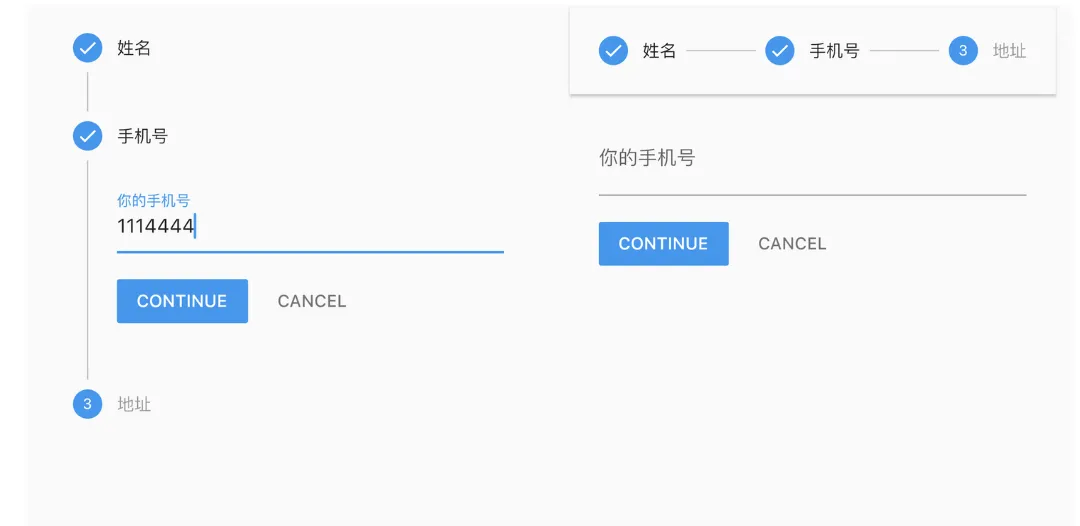
按照步骤提交信息完整例子
import 'package:flutter/material.dart';
class GRStepperPage extends StatefulWidget {
const GRStepperPage({Key? key}) : super(key: key);
@override
State<GRStepperPage> createState() => _GRStepperPageState();
}
class _GRStepperPageState extends State<GRStepperPage> {
//当前step
int _currentStep = 0;
TextEditingController name = TextEditingController();
TextEditingController phone = TextEditingController();
TextEditingController email = TextEditingController();
TextEditingController address = TextEditingController();
List<Step> stepList() => [
Step(
state: _currentStep <= 0 ? StepState.editing : StepState.complete,
isActive: _currentStep >= 0,
title: const Text('账号'),
content: Container(
child: Column(
children: [
const SizedBox(height: 8.0),
TextField(
controller: name,
decoration: const InputDecoration(
border: OutlineInputBorder(),
labelText: '名字'
),
),
const SizedBox(height: 8.0),
TextField(
controller: phone,
decoration: const InputDecoration(
border: OutlineInputBorder(),
labelText: '手机号'
),
),
],
),
)
),
Step(
state: _currentStep <= 1 ? StepState.editing : StepState.complete,
isActive: _currentStep >= 1,
title: const Text('邮箱/地址'),
content: Container(
child: Column(
children: [
const SizedBox(height: 8.0),
TextField(
controller: email,
decoration: const InputDecoration(
border: OutlineInputBorder(),
labelText: '邮箱'
),
),
const SizedBox(height: 8.0),
TextField(
controller: address,
decoration: const InputDecoration(
border: OutlineInputBorder(),
labelText: '地址'
),
)
],
),
)
),
Step(
state: StepState.complete,
isActive: _currentStep >= 2,
title: const Text('提交'),
content: Container(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('姓名:${name.text}'),
Text('手机号:${phone.text}'),
Text('邮箱:${email.text}'),
Text('邮箱:${address.text}'),
],
),
)
)
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('步骤条'),),
body: Padding(
padding: const EdgeInsets.all(15.0),
child: Column(
children: [
Expanded(
child: Stepper(
type: StepperType.horizontal,
currentStep: _currentStep,
steps: stepList(),
controlsBuilder: (BuildContext context,ControlsDetails details){
return Row(
mainAxisAlignment: _currentStep != 0
? MainAxisAlignment.spaceBetween
: MainAxisAlignment.end,
children: [
if(_currentStep != 0)
TextButton(onPressed: details.onStepCancel, child: const Text('上一步')),
TextButton(onPressed: details.onStepContinue, child: const Text('下一步'))
],
);
},
onStepTapped: (int index){
setState(()=> _currentStep -= 1);
},
onStepContinue: (){
if(_currentStep < (stepList().length -1)){
setState(()=> _currentStep += 1);
}
},
onStepCancel: (){
if(_currentStep == 0){
return;
}
setState(() => _currentStep -= 1);
},
),
)
],
),
),
);
}
}
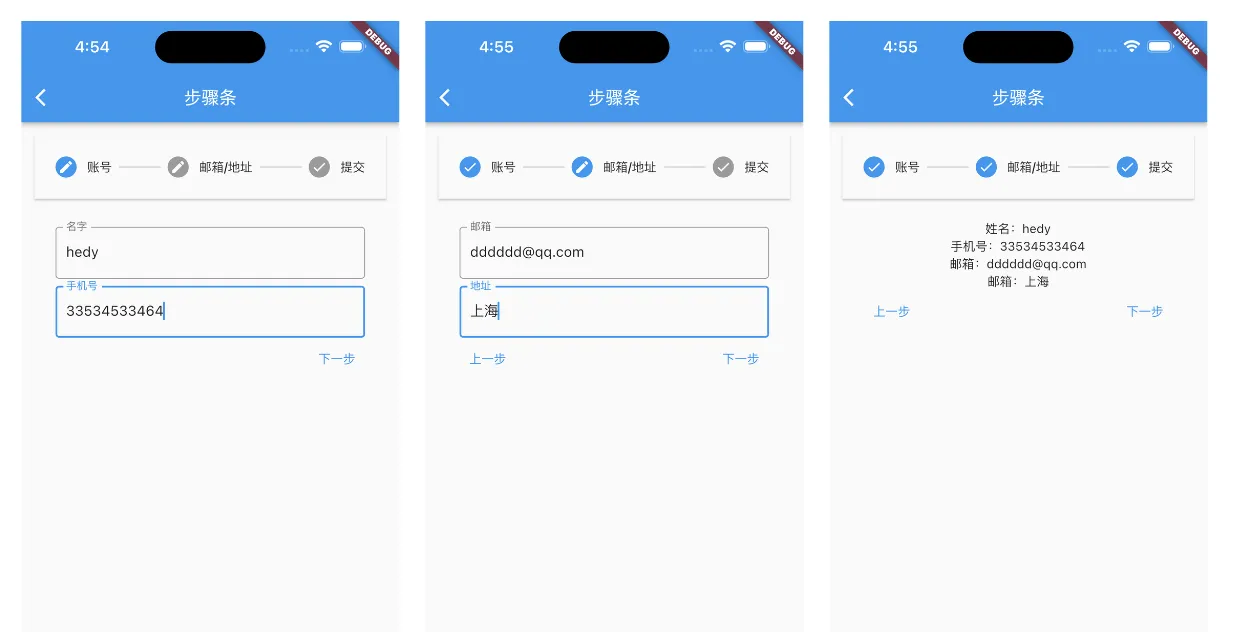
评论(0)