知识点
CustomPaint
CustomPainter
类
Paint()
Path()
drawPath
import 'dart:math';
import 'dart:ui';
import 'package:flutter/material.dart';
class CustomPaintPage extends StatefulWidget {
const CustomPaintPage({Key? key}) : super(key: key);
@override
State<CustomPaintPage> createState() => _CustomPaintPageState();
}
class _CustomPaintPageState extends State<CustomPaintPage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('CustomPaint'),),
body: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
const SizedBox(height: 30,),
CustomPaint(
size: const Size(250, 230),
painter: MyPainterHeart(Colors.red,Colors.transparent,0.0),
),
CustomPaint(
size: const Size(200, 160),
painter: MyPainterHeart(Colors.red,Colors.green,10.0),
),
CustomPaint(
size: const Size(200, 240),
painter: MyPainterHeart(Colors.green,Colors.black,1.0),
),
],
),
),
);
}
}
class MyPainterHeart extends CustomPainter{
//爱心颜色
final Color bodyColor;
//爱心边框颜色
final Color borderColor;
//边框宽度
final double borderWidth;
MyPainterHeart(
this.bodyColor,
this.borderColor,
this.borderWidth,
);
@override
void paint(Canvas canvas, Size size) {
final Paint body = Paint();
body
..color = bodyColor
..style = PaintingStyle.fill
..strokeWidth = 0;
final Paint border = Paint();
border
..color = borderColor
..style = PaintingStyle.stroke
..strokeCap = StrokeCap.round
..strokeWidth = borderWidth;
final double w = size.width;
final double h = size.height;
final Path path = Path();
path
..moveTo(0.5*w, 0.4*h)
..cubicTo(0.2*w, 0.1*h, -0.25*w, 0.6*h, 0.5*w, h)
..moveTo(0.5*w, 0.4*h)
..cubicTo(0.8*w, 0.1*h, 1.25*w, 0.6*h, 0.5*w, h);
canvas.drawPath(path, body);
canvas.drawPath(path, border);
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) {
// TODO: implement shouldRepaint
//throw UnimplementedError();
return true;
}
}
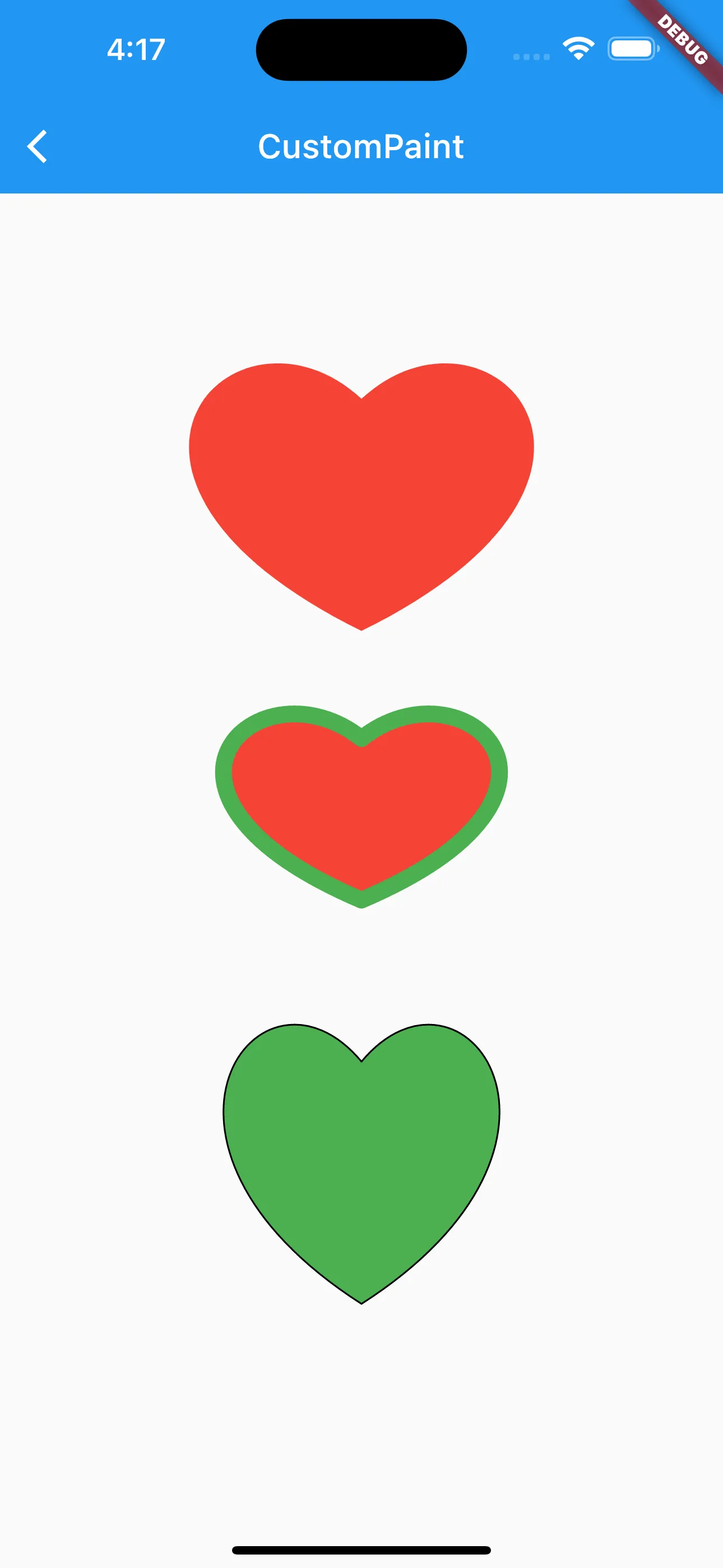