知识点
CustomPaint
CustomPainter
类
Paint
drawRect
drawCircle
import 'dart:math';
import 'dart:ui';
import 'package:flutter/material.dart';
class CustomPaintPage extends StatefulWidget {
const CustomPaintPage({Key? key}) : super(key: key);
@override
State<CustomPaintPage> createState() => _CustomPaintPageState();
}
class _CustomPaintPageState extends State<CustomPaintPage> {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.black26,
appBar: AppBar(title: const Text('CustomPaint'),),
body: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
const SizedBox(height: 30,),
CustomPaint(
painter: MyPainter4(),
size: const Size(400,400),
),
],
),
),
);
}
}
//五彩棋盘
class MyPainter4 extends CustomPainter{
@override
void paint(Canvas canvas,Size size){
var rect = Offset.zero & size;
drawChessboard(canvas, rect);
drawPieces(canvas, rect);
}
//棋盘
void drawChessboard(Canvas canvas, Rect rect){
var paint = Paint()
..isAntiAlias = true
..strokeWidth = 1.0
..style = PaintingStyle.stroke
..invertColors = false;
double w = rect.width/15;
double h = rect.height/15;
for(int i = 0; i < 15; i++){
for(int j = 0; j < 15; j++){
Rect rect = Rect.fromPoints(Offset(w * j,h * i), Offset(w*(j+1),h*(i+1)));
switch(i){
case 0:
case 8:
case 6:
case 10:
case 12:
case 14:
canvas.drawRect(rect, paint..color = (i%2==0 && j%2==0 ? Colors.blue : Colors.deepOrange));
break;
case 1:
case 3:
case 5:
case 9:
case 11:
canvas.drawRect(rect, paint..color = (i%3==1 && j%3==1 ? Colors.red : Colors.green));
break;
case 2:
case 7:
case 4:
case 13:
canvas.drawRect(rect, paint..color = (i%2==0 && j%2==0 ? Colors.amber : Colors.purple));
break;
}
}
}
}
//棋子
void drawPieces(Canvas canvas,Rect rect){
double w = rect.width/15;
double h = rect.height/15;
var paint = Paint()
..style = PaintingStyle.fill
..color = Colors.green;
canvas.drawCircle(
Offset(rect.center.dx - w/2, rect.center.dy - h/2),
min(w/2, h/2) - 2,
paint
);
paint.color = Colors.white;
canvas.drawCircle(
Offset(rect.center.dx + w/2, rect.center.dy + h/2),
min(w/2, h/2) - 2,
paint
);
canvas.drawCircle(
Offset(1, rect.center.dy + h/2),
min(w/2, h/2) - 2,
paint
);
}
@override
bool shouldRepaint(MyPainter oldDelegate){
//shouldRepaint方法通常在当前实例和旧实例属性不一致时返回true
return true;
}
}
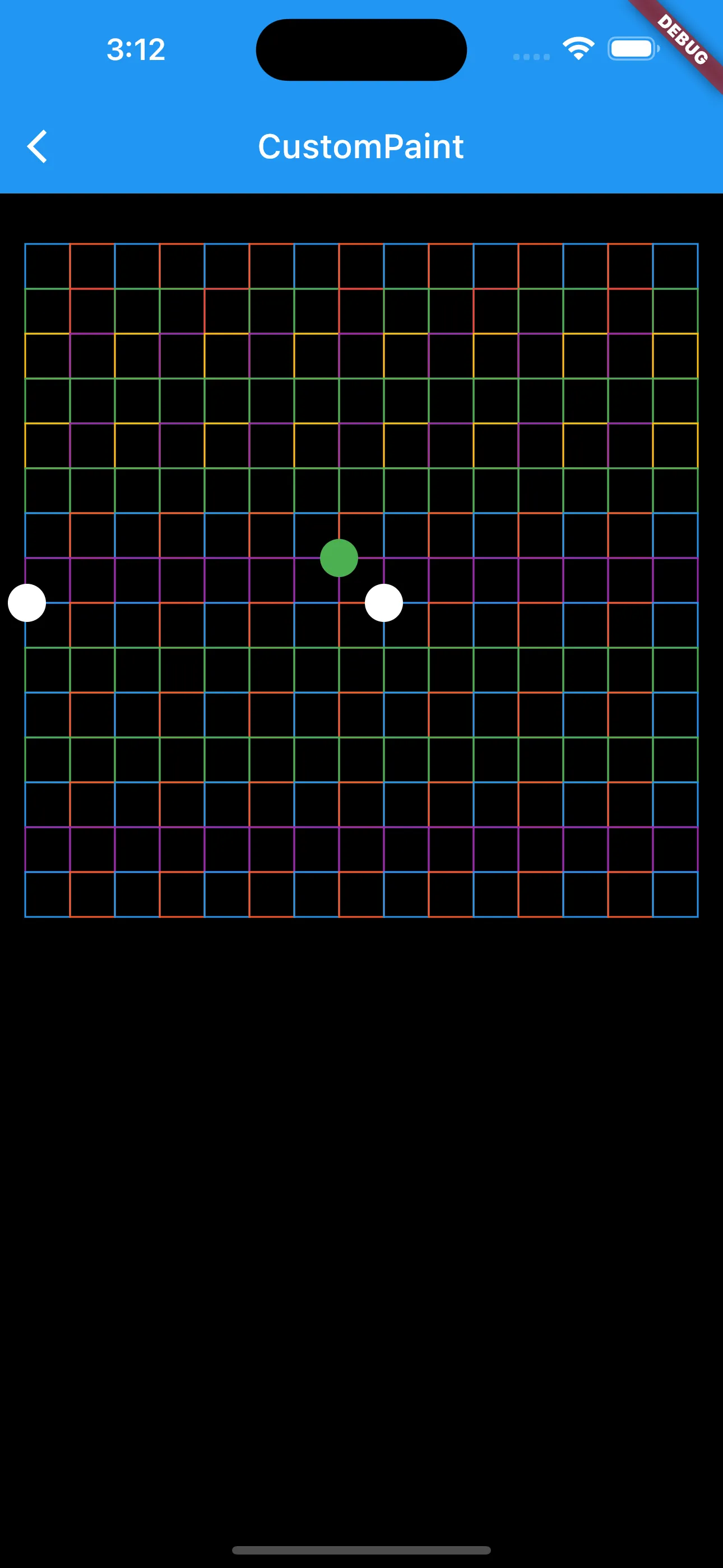